HTML Tutorial
Creating a comprehensive HTML tutorial involves covering various aspects of HTML, including its fundamental elements, attributes, and features. Here’s a detailed guide that incorporates the topics we've discussed:
HTML Tutorial
1. Introduction to HTML
HTML (HyperText Markup Language) is the standard language used to create and design web pages. It structures content on the web and provides a way to format text, add images, create links, and more.
2. HTML Editors
HTML editors are tools used to write and edit HTML code. They come in various forms, from simple text editors to specialized IDEs (Integrated Development Environments) with features like syntax highlighting and auto-completion.
Examples of HTML Editors:
- Text Editors: Notepad, Sublime Text
- IDE: Visual Studio Code, Atom
3. HTML Basics
HTML documents are composed of elements that include tags, attributes, and content. A basic HTML document includes the <!DOCTYPE html>
, <html>
, <head>
, and <body>
tags.
Basic HTML Document Example:
<!DOCTYPE html>
<html>
<head>
<title>My First HTML Page</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is a paragraph of text.</p>
</body>
</html>
4. HTML Elements
HTML elements are the building blocks of web pages. They are defined by tags and can include attributes and content.
Common HTML Elements:
- Headings:
<h1>
,<h2>
, etc. - Paragraphs:
<p>
- Links:
<a>
- Images:
<img>
5. HTML Attributes
Attributes provide additional information about HTML elements. They are specified in the opening tag and usually come in name/value pairs.
Example:
<a href="https://www.example.com" target="_blank">Visit Example</a>
<img src="image.jpg" alt="Description">
6. HTML Headings
Headings are used to define the importance and hierarchy of content. HTML provides six levels of headings, from <h1>
to <h6>
.
Example:
<h1>Main Heading</h1>
<h2>Subheading</h2>
<h3>Sub-subheading</h3>
7. HTML Paragraphs
The <p>
tag defines paragraphs in HTML. It automatically adds space before and after the content.
Example:
<p>This is a paragraph of text.</p>
<p>This is another paragraph.</p>
8. HTML Styles
CSS (Cascading Style Sheets) is used to style HTML elements. Styles can be applied inline, internally, or externally.
Inline Style Example:
<p style="color: blue;">This is a blue paragraph.</p>
Internal Style Example:
<head>
<style>
p {
color: blue;
}
</style>
</head>
External Style Example:
<link rel="stylesheet" href="styles.css">
9. HTML Formatting
HTML provides tags to format text, such as bold, italic, and underline.
Example:
<b>Bold text</b>
<i>Italic text</i>
<u>Underlined text</u>
10. HTML Quotations
Quotations are used to include text from other sources. HTML offers several tags for this purpose.
Example:
<blockquote cite="https://www.example.com">
This is a blockquote from an external source.
</blockquote>
<cite>Author Name</cite>
11. HTML Comments
Comments are used to add notes or explanations within the HTML code. They are not displayed in the browser.
Example:
<!-- This is a comment -->
<p>This is visible text.</p>
12. HTML Colors
Colors can be applied to text, backgrounds, borders, and more using CSS.
Example:
<p style="color: red; background-color: yellow;">This text is red with a yellow background.</p>
13. HTML CSS
CSS (Cascading Style Sheets) is used to style HTML elements. It can be included inline, internally, or externally.
Example:
<style>
body {
font-family: Arial, sans-serif;
}
</style>
14. HTML Links
Links allow users to navigate between pages or to external sites. The <a>
tag is used to create links.
Example:
<a href="https://www.example.com">Visit Example</a>
15. HTML Images
Images can be added using the <img>
tag. The src
attribute specifies the image source, and alt
provides alternative text.
Example:
<img src="image.jpg" alt="Description of the image">
16. HTML Favicon
A favicon is a small icon displayed in the browser tab. It is linked in the <head>
section.
Example:
<link rel="icon" href="favicon.ico" type="image/x-icon">
17. HTML Page Title
The page title is displayed in the browser tab and is set using the <title>
tag in the <head>
section.
Example:
<head>
<title>My Page Title</title>
</head>
18. HTML Tables
Tables are used to organize data into rows and columns. They are created using <table>
, <tr>
, <th>
, and <td>
tags.
Example:
<table>
<tr>
<th>Header 1</th>
<th>Header 2</th>
</tr>
<tr>
<td>Row 1, Cell 1</td>
<td>Row 1, Cell 2</td>
</tr>
</table>
19. HTML Lists
Lists can be ordered (<ol>
) or unordered (<ul>
). List items are created with <li>
.
Example:
Unordered List:
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
Ordered List:
<ol>
<li>First</li>
<li>Second</li>
</ol>
20. HTML Block & Inline
HTML elements are either block-level (e.g., <div>
, <p>
) or inline (e.g., <span>
, <a>
). Block-level elements take up the full width available, while inline elements take up only as much width as necessary.
Example:
<div>This is a block-level element.</div>
<span>This is an inline element.</span>
21. HTML Div
The <div>
tag is used as a container for other HTML elements. It is often used for layout purposes.
Example:
<div class="container">
<p>This is inside a div.</p>
</div>
22. HTML Classes
Classes are used to apply styles to multiple elements. They are defined with the class
attribute.
Example:
<style>
.highlight {
background-color: yellow;
}
</style>
<p class="highlight">This text is highlighted.</p>
23. HTML Id
The id
attribute is used to uniquely identify an element. It must be unique within a document.
Example:
<p id="unique">This paragraph has a unique ID.</p>
24. HTML Iframes
The <iframe>
tag is used to embed another HTML page within the current page.
Example:
<iframe src="https://www.example.com" width="600" height="400"></iframe>
25. HTML JavaScript
JavaScript can be embedded within HTML to add interactivity. It is included using the <script>
tag.
Example:
<script>
function greet() {
alert('Hello, World!');
}
</script>
<button onclick="greet()">Click me</button>
26. HTML File Paths
File paths specify the location of resources like images, stylesheets, and scripts. They can be absolute or relative.
Example:
<img src="images/photo.jpg" alt="Photo">
<link rel="stylesheet" href="styles/main.css">
27. HTML Head
The <head>
section contains meta-information about the HTML document, such as links to stylesheets and scripts.
Example:
<head>
<title>My Page</title>
<meta charset="UTF-8">
<link rel="stylesheet" href="styles.css">
</head>
28. HTML Layout
HTML layout involves structuring the content of a webpage using various HTML elements and CSS for styling.
Example:
<body>
<header>Header Content</header>
<nav>Navigation Links</nav>
<main>Main Content</main>
<footer>Footer Content</footer>
</body>
29. HTML Responsive
Responsive design ensures that web pages look good on all devices by using flexible layouts, images, and media queries.
Example:
<style>
@media (max-width: 600px) {
.container {
width: 100%;
}
}
</style>
30. HTML Computercode
The <code>
tag is used to display a single line of code, while <pre>
is used for preformatted text.
Example:
<pre>
<code>
const greet = () => {
console.log('Hello, World!');
};
</code>
</pre>
31. HTML Semantics
Semantic HTML elements clearly describe their meaning in a human- and machine-readable way. Examples include <article>
, <section>
, and <footer>
.
Example:
<article>
<h1>Article Title</h1>
<p>Article content.</p>
</article>
32. HTML Style Guide
An HTML style guide ensures consistent and readable code. It covers naming conventions, indentation, and organization.
Example:
- Use lowercase for element names and attribute names.
- Use double quotes for attribute values.
- Indent nested elements with two spaces.
33. HTML Entities
HTML entities represent special characters that might otherwise be interpreted as HTML code.
Example:
<p>© 2024 My Website</p>
34. HTML Symbols
Symbols can be included using HTML entities or Unicode characters.
Example:
<p>☀ Sunny Weather</p>
35. HTML Emojis
Emojis can be included in HTML using Unicode characters.
Example:
<p>😊 🌍</p>
36. HTML Charsets
Charset specifies the character encoding for HTML documents. The most common is UTF-8.
Example:
<meta charset="UTF-8">
37. HTML URL Encode
URL encoding converts special characters into a format that can be transmitted over the internet.
Example:
<a href="search?q=html%20tutorial">Search for HTML Tutorial</a>
38. HTML vs. XHTML
- HTML: A flexible markup language for web pages.
- XHTML: A stricter version of HTML that adheres to XML rules.
39. HTML Forms
HTML forms collect user input. They are defined using the <form>
tag and can include various input elements like text fields, checkboxes, and submit buttons.
Example:
<form action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<input type="submit" value="Submit">
</form>
40. HTML Form Attributes
Form attributes define the behavior of the form and its elements, such as action
, method
, and name
.
Example:
<form action="/submit" method="post">
<input type="text" name="username" required>
</form>
41. HTML Form Elements
Form elements include <input>
, <select>
, <textarea>
, and <button>
. They are used to create different types of user inputs.
Example:
<form>
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<label for="bio">Bio:</label>
<textarea id="bio" name="bio"></textarea>
<input type="submit" value="Submit">
</form>
42. HTML Input Types
Different input types are available for various types of user data, such as text, password, email, and file.
Example:
<form>
<input type="text" name="username" placeholder="Username">
<input type="password" name="password" placeholder="Password">
<input type="email" name="email" placeholder="Email">
<input type="file" name="file">
<input type="submit" value="Submit">
</form>
43. HTML Input Attributes
Input attributes modify the behavior and validation of form fields, such as placeholder
, required
, and maxlength
.
Example:
<input type="text" name="username" placeholder="Enter your username" required>
44. Input Form Attributes
Attributes like action
, method
, and enctype
determine how form data is submitted and processed.
Example:
<form action="/submit" method="post" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="Upload">
</form>
45. HTML Media
HTML supports embedding media elements like audio and video using <audio>
and <video>
tags.
Example:
<audio controls>
<source src="audio.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
<video width="320" height="240" controls>
<source src="video.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
46. HTML Canvas
The <canvas>
element is used to draw graphics on the fly via JavaScript.
Example:
<canvas id="myCanvas" width="200" height="100"></canvas>
<script>
var canvas = document.getElementById('myCanvas');
var context = canvas.getContext('2d');
context.fillStyle = 'red';
context.fillRect(10, 10, 150, 75);
</script>
47. HTML SVG
SVG (Scalable Vector Graphics) allows for the creation of vector-based graphics that are scalable and resolution-independent.
Example:
<svg width="100" height="100">
<circle cx="50" cy="50" r="40" stroke="black" stroke-width="3" fill="red" />
</svg>
48. HTML Audio
The <audio>
tag is used to play audio files.
Example:
<audio controls>
<source src="audio.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
49. HTML Plug-ins
HTML plug-ins extend the functionality of web pages. Examples include Flash and Java applets, though they are less common today due to security concerns.
50. HTML YouTube
To embed a YouTube video, use the <iframe>
tag with the video's URL.
Example:
<iframe width="560" height="315" src="https://www.youtube.com/embed/dQw4w9WgXcQ" frameborder="0" allowfullscreen></iframe>
51. HTML APIs
HTML APIs (Application Programming Interfaces) allow for advanced functionalities, such as geolocation and local storage.
Example:
<button onclick="getLocation()">Get Location</button>
<script>
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
alert("Geolocation is not supported by this browser.");
}
}
function showPosition(position) {
alert("Latitude: " + position.coords.latitude +
"\nLongitude: " + position.coords.longitude);
}
</script>
52. HTML Drag/Drop
HTML5 provides a drag-and-drop API for moving elements.
Example:
<div id="drag1" draggable="true" ondragstart="drag(event)">Drag me</div>
<div id="drop1" ondrop="drop(event)" ondragover="allowDrop(event)">Drop here</div>
<script>
function allowDrop(ev) {
ev.preventDefault();
}
function drag(ev) {
ev.dataTransfer.setData("text", ev.target.id);
}
function drop(ev) {
ev.preventDefault();
var data = ev.dataTransfer.getData("text");
var draggedElement = document.getElementById(data);
ev.target.appendChild(draggedElement);
}
</script>
53. HTML Geolocation
HTML5's Geolocation API allows web pages to access the user's location.
Example:
<button onclick="getLocation()">Get Location</button>
<script>
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
alert("Geolocation is not supported by this browser.");
}
}
function showPosition(position) {
alert("Latitude: " + position.coords.latitude +
"\nLongitude: " + position.coords.longitude);
}
</script>
54. HTML Web Storage
Web Storage provides a way to store data locally in the user's browser using localStorage
and sessionStorage
.
Example:
<button onclick="saveData()">Save Data</button>
<button onclick="loadData()">Load Data</button>
<script>
function saveData() {
localStorage.setItem("name", "John Doe");
}
function loadData() {
alert(localStorage.getItem("name"));
}
</script>
55. HTML Web Workers
Web Workers allow JavaScript to run in the background, without affecting the performance of the web page.
Example:
<script>
if (window.Worker) {
var myWorker = new Worker("worker.js");
myWorker.onmessage = function(e) {
console.log('Message received from worker: ', e.data);
};
myWorker.postMessage('Hello, Worker!');
}
</script>
56. HTML SSE
Server-Sent Events (SSE) allow servers to push updates to the web page.
Example:
<script>
var eventSource = new EventSource('events.php');
eventSource.onmessage = function(event) {
console.log('New message: ', event.data);
};
</script>
57. HTML Examples
Examples help illustrate various HTML concepts and techniques.
Example:
<!DOCTYPE html>
<html>
<head>
<title>Sample Page</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a sample page to demonstrate HTML basics.</p>
</body>
</html>
58. HTML Website
Creating a complete website involves using HTML along with CSS for styling and JavaScript for functionality.
59. HTML Certificate
Various online platforms offer HTML certificates upon completion of courses, validating your skills and knowledge in HTML.
60. HTML Bootcamp
HTML bootcamps provide intensive, short-term training to help individuals learn HTML and related web technologies quickly.
61. HTML Accessibility
Accessibility ensures that web content is usable by people with disabilities. This includes proper use of semantic HTML, ARIA attributes, and keyboard navigation.
Example:
<a href="#content" accesskey="1">Skip to content</a>
62. HTML Summary
HTML is a fundamental technology for web development, enabling the creation and structuring of web content. Understanding its elements, attributes, and capabilities is essential for building effective web pages.
63. HTML References
References include official documentation, tutorials, and resources for further learning and exploration of HTML.
References:
64. HTML Tag List
A list of all HTML tags, including their purposes and usage.
65. HTML Attributes
Attributes provide additional information about HTML elements and modify their behavior.
66. HTML Global Attributes
Global attributes apply to all HTML elements and include id
, class
, style
, etc.
67. HTML Browser Support
Different browsers may support HTML features differently. Checking browser compatibility is crucial for ensuring consistent user experiences.
68. HTML Events
Events are actions that occur in the browser, such as clicks and key presses. JavaScript can be used to handle these events.
Example:
<button onclick="alert('Button clicked!')">Click me</button>
69. HTML Audio/Video
Handling audio and video content in HTML involves using the <audio>
and <video>
elements to embed media.
70. HTML Doctypes
The <!DOCTYPE>
declaration defines the document type and version of HTML being used.
Example:
<!DOCTYPE html>
71. HTML Character Sets
Character sets determine how characters are encoded in HTML documents. UTF-8 is the most commonly used charset.
72. HTML URL Encode
URL encoding converts special characters in URLs into a format that can be transmitted over the internet.
Example:
<a href="search?q=html%20tutorial">Search for HTML Tutorial</a>
73. HTML Lang Codes
Language codes specify the language of the document, helping search engines and accessibility tools.
Example:
<html lang="en">
74. HTTP Messages
HTTP messages are used for communication between clients and servers, including requests and responses.
Example:
- Request:
GET /index.html HTTP/1.1
- Response:
HTTP/1.1 200 OK
75. HTTP Methods
HTTP methods define the action to be performed on a resource, such as GET
, POST
, PUT
, and DELETE
.
Example:
- GET: Retrieve data
- POST: Submit data
76. PX to EM Converter
Converting pixels to em units involves understanding the base font size. A common formula is: 1em = font-size
.
Example:
/* Assuming base font size is 16px */
.element {
font-size: 1.5em; /* 24px */
}
77. Keyboard Shortcuts
Keyboard shortcuts are used to perform actions quickly.
Examples:
- Ctrl + C: Copy
- Ctrl + V: Paste
This guide should give you a solid understanding of HTML and its various components. If you have any specific questions or need further details on any topic, feel free to ask!
Web Technology View More
Learn Ansible
Ansible is an open-source automation tool that sim..
Learn HTML
HTML5 supports multimedia elements such as audio a..
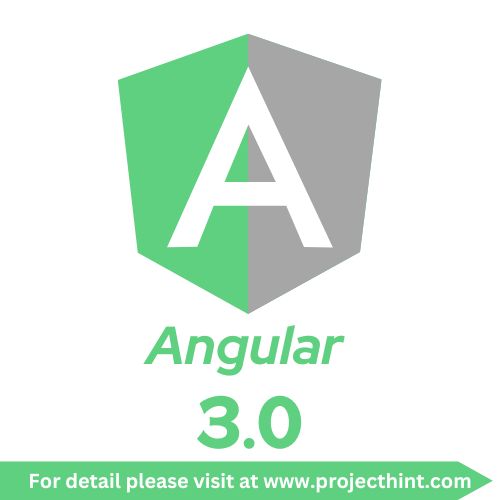
Learn Angular 3
Angular..
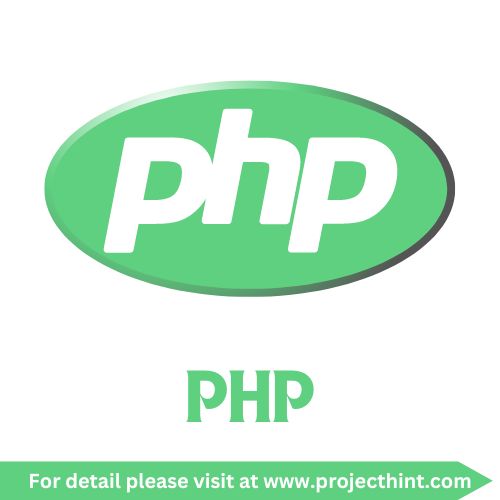
Learn PHP
Introduction to PHP: A Powerful Scripting Language..
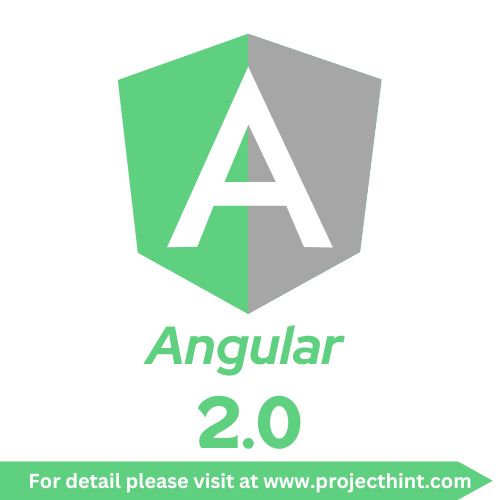
Learn Angular 2.0
Angular 2..
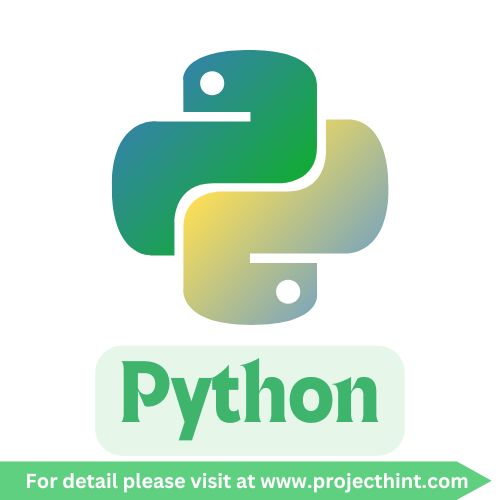
Learn Python
Python Tutorials..
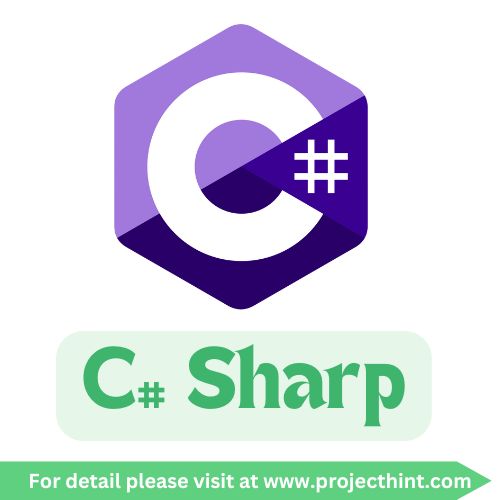
Learn C# Sharp
C# Syntax - Learn the basic syntax of C# programmi..
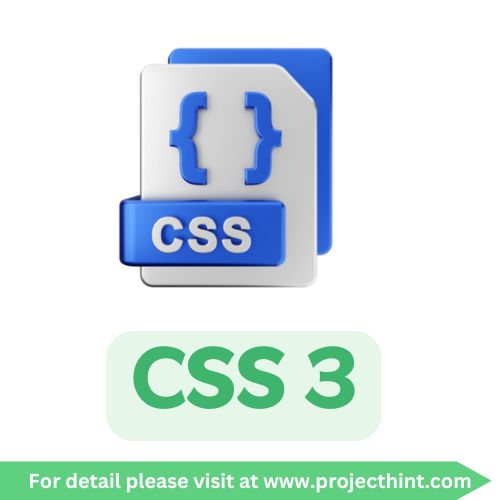
Learn CSS
What is CSS?..
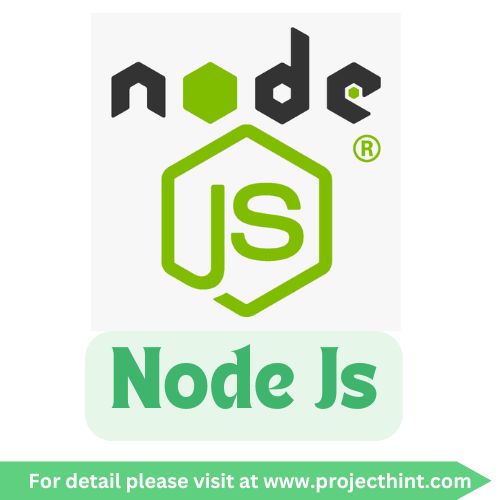
Learn Node Js
Node.js: A Powerful JavaScript Runtime for Server-..
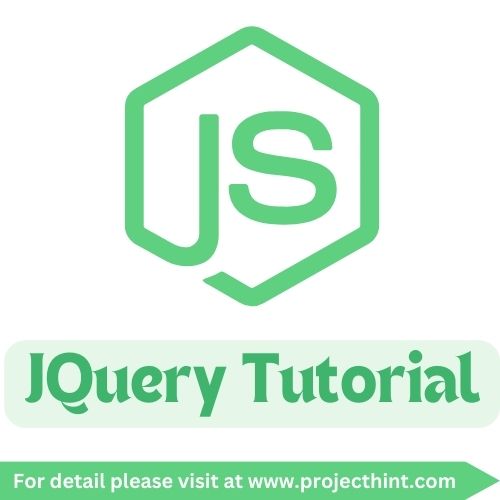
Learn JQuery Framework
jQuery is a JavaScript library designed to simplif..