NodeJs Tutorial
Node.js Tutorial: Getting Started with Server-Side JavaScript
Node.js is a powerful platform built on Chrome's V8 JavaScript engine, allowing you to execute JavaScript on the server. It is designed to build scalable, fast, and efficient web applications, handling multiple requests simultaneously without blocking the main thread. This tutorial will guide you through the fundamentals of Node.js, from installation to creating a simple server.
1. Installing Node.js and NPM
To get started, you’ll need to install Node.js on your machine. Node.js comes with NPM (Node Package Manager), which helps you manage packages and libraries for your projects.
Steps:
- Windows / macOS / Linux:
- Visit the Node.js website and download the latest stable version.
- Run the installer and follow the instructions.
- To verify the installation, open a terminal or command prompt and run the following commands:
bash
node -v npm -v
- You should see the version numbers of both Node.js and NPM.
2. Creating Your First Node.js Application
Let’s start by creating a simple Node.js application that sets up a basic HTTP server and responds with "Hello, World!".
Steps:
-
Create a new directory for your project:
bashmkdir my-first-node-app cd my-first-node-app
-
Initialize a Node.js project:
bashnpm init -y
This command generates a
package.json
file, which contains information about your project and its dependencies. -
Create a new file named
app.js
in your project folder. -
Write the following code inside
app.js
to create an HTTP server:// Import the HTTP module const http = require('http'); // Create a server object const server = http.createServer((req, res) => { res.writeHead(200, { 'Content-Type': 'text/html' }); res.write('Hello, World!'); res.end(); }); // The server listens on port 3000 server.listen(3000, () => { console.log('Server running on http://localhost:3000'); });
-
To run the server, use the following command:
bashnode app.js
-
Open your browser and navigate to
http://localhost:3000
. You should see the message "Hello, World!".
3. Understanding Modules and NPM
Node.js has a modular architecture, and you can import different modules (either built-in or third-party) to extend its functionality.
Built-In Modules:
fs
: File system operationshttp
: Create HTTP serverspath
: Handle file pathsos
: Get information about the operating system
Here’s an example of reading a file with the fs
module:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Using NPM Packages:
You can install third-party modules using NPM. For example, to install the popular express
framework, you can run:
npm install express
Here’s how to create a basic Express app:
const express = require('express');
const app = express();
// Define a route
app.get('/', (req, res) => {
res.send('Hello from Express!');
});
// Start the server
app.listen(3000, () => {
console.log('Express server running on http://localhost:3000');
});
4. Handling HTTP Requests
Node.js can handle various HTTP methods like GET, POST, PUT, and DELETE. Let’s explore how to handle GET and POST requests.
GET Request:
const http = require('http');
const url = require('url');
http.createServer((req, res) => {
const queryObject = url.parse(req.url, true).query;
res.writeHead(200, { 'Content-Type': 'text/html' });
res.end(`Hello, ${queryObject.name || 'Guest'}!`);
}).listen(3000);
When you visit http://localhost:3000/?name=John
, it will respond with "Hello, John!".
POST Request:
const http = require('http');
http.createServer((req, res) => {
if (req.method === 'POST') {
let body = '';
req.on('data', chunk => {
body += chunk.toString();
});
req.on('end', () => {
res.writeHead(200, { 'Content-Type': 'text/html' });
res.end(`Received data: ${body}`);
});
}
}).listen(3000);
You can use tools like Postman or curl to test this POST request:
curl -X POST -d "name=John" http://localhost:3000
5. Working with the File System
Node.js allows you to interact with the file system to read, write, or delete files using the built-in fs
module.
Reading a File:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Writing to a File:
const fs = require('fs');
fs.writeFile('output.txt', 'Hello, Node.js!', (err) => {
if (err) throw err;
console.log('File has been saved!');
});
6. Node.js with Databases
Node.js can interact with various databases like MySQL, MongoDB, or PostgreSQL. Let’s look at an example using MongoDB with the mongoose
package.
Steps:
-
Install
mongoose
:bashnpm install mongoose
-
Connect to a MongoDB database:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true });
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', () => {
console.log('Connected to MongoDB');
});
- Define a schema and a model:
const userSchema = new mongoose.Schema({
name: String,
age: Number
});
const User = mongoose.model('User', userSchema);
// Create a new user
const user = new User({ name: 'John', age: 30 });
user.save().then(() => console.log('User saved!'));
7. Conclusion
Node.js is an incredibly powerful tool for building fast and scalable web applications. It allows you to use JavaScript on the server-side, making full-stack development more streamlined. With an active ecosystem and vast library of modules, Node.js is an excellent choice for developing everything from RESTful APIs to real-time applications like chat apps.
As you continue learning Node.js, explore more advanced topics such as:
- Working with WebSockets for real-time communication
- Using asynchronous programming (promises, async/await)
- Building RESTful APIs with Express
- Implementing authentication and authorization
This tutorial should have given you a good foundation to get started building applications in Node.js.
Web Technology View More
Learn Ansible
Ansible is an open-source automation tool that sim..
Learn HTML
HTML5 supports multimedia elements such as audio a..
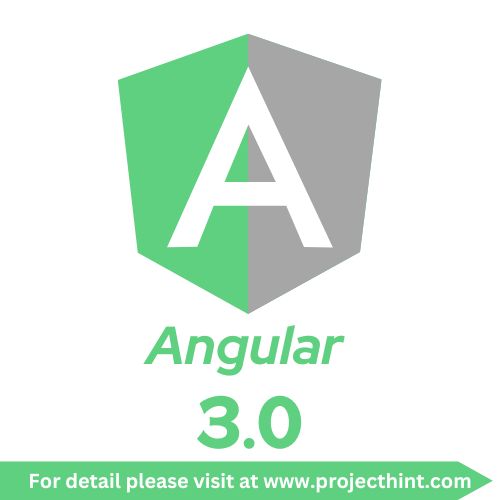
Learn Angular 3
Angular..
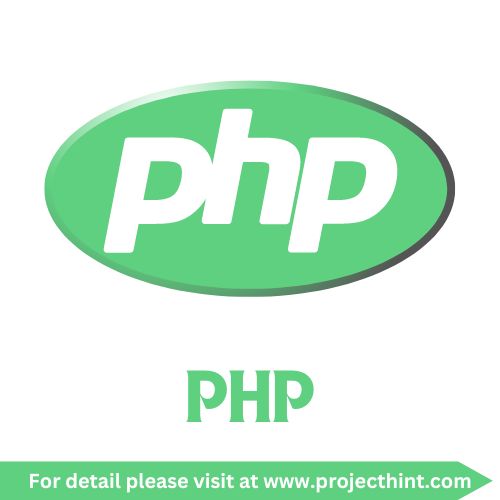
Learn PHP
Introduction to PHP: A Powerful Scripting Language..
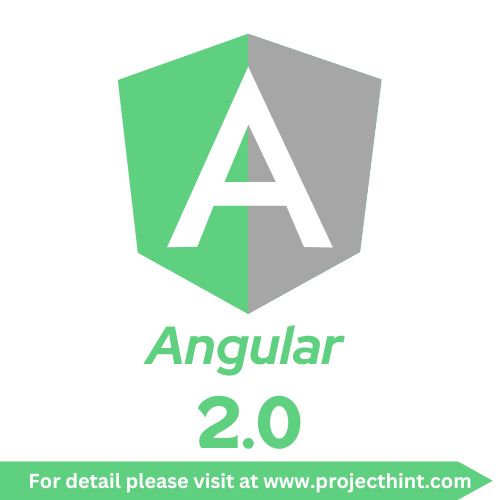
Learn Angular 2.0
Angular 2..
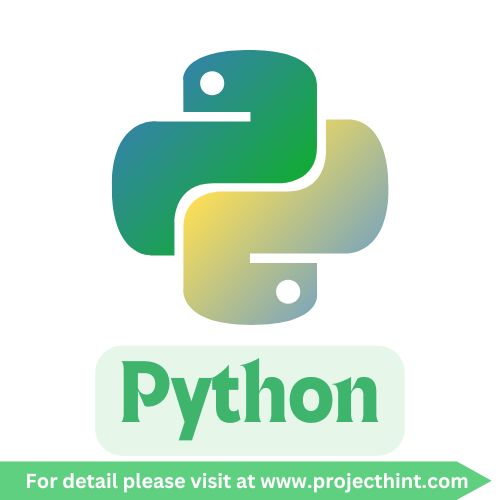
Learn Python
Python Tutorials..
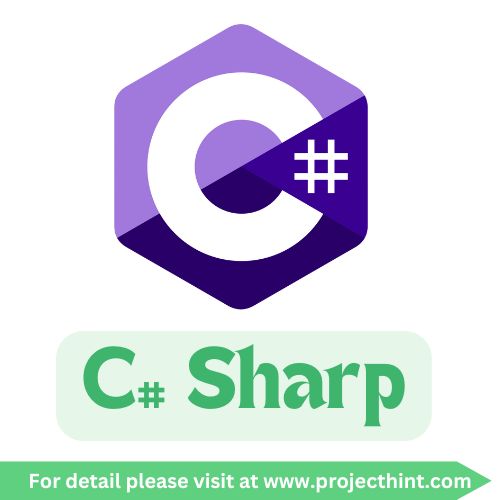
Learn C# Sharp
C# Syntax - Learn the basic syntax of C# programmi..
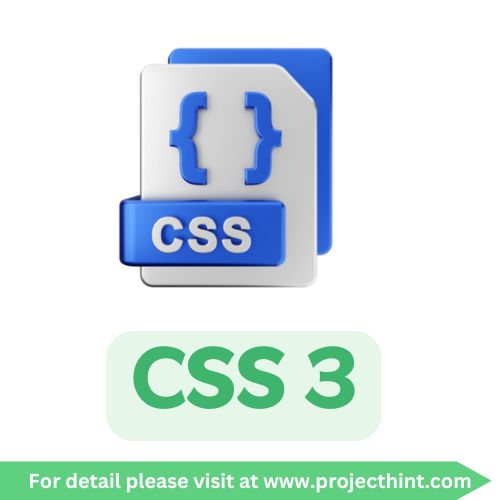
Learn CSS
What is CSS?..
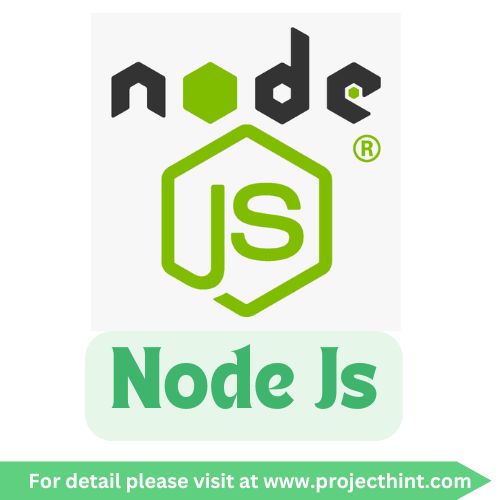
Learn Node Js
Node.js: A Powerful JavaScript Runtime for Server-..